日常使用单行的时候都是以列表的形式,如横向或者竖向,而一但需要多行显示的时候就不行了,如下面的效果图:
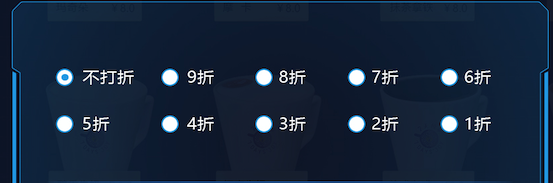
实现这样的效果图有以下几种思路:
- 使用
RadioGroup
竖向布局。中间嵌套LinearLayout
来使用。但是这样RadioGroup
会失去单选的功能,因为所有的RadioButton
必须作为RadioGroup
的子view才行。
- 分2行
RadioGroup
。使用RadioGroup
的setOnCheckChangeListener
来监听,当选中的radio是属于group1的时候,使用group2的clearCheck()
方法清空group2的状态,反之亦然。但是由于使用clearCheck()
方法时也会回调checkChange()
方法,所以方法执行时无法判断是选中时回调的方法还是清空时回调的方法
- 重写
RadioGroup
。让其支持多行显示,但是这样太复杂了。
- 使用
RadioButton
数组。不使用RadioGroup
的setOnCheckChange
方法,使用RadioButton
的setOnCheckChange
方法来手动管理radio的状态
我比较倾向第4种,以下是实现方式:
.xml文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| <RadioGroup android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/rg_firstLine" android:layout_marginTop="@dimen/margin_xxlarge" android:orientation="horizontal"> <RadioButton style="@style/discount" android:id="@+id/rb_discount0" android:checked="true" android:layout_marginStart="@dimen/margin_xxlarge" android:text="@string/label_no_discount" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount1" android:text="@string/label_discount1" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount2" android:text="@string/label_discount2" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount3" android:text="@string/label_discount3" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount4" android:text="@string/label_discount4" /> </RadioGroup>
<RadioGroup android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/rg_secondLine" android:layout_marginTop="@dimen/margin_large" android:orientation="horizontal"> <RadioButton style="@style/discount" android:id="@+id/rb_discount5" android:layout_marginStart="@dimen/margin_xxlarge" android:text="@string/label_discount5" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount6" android:text="@string/label_discount6" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount7" android:text="@string/label_discount7" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount8" android:text="@string/label_discount8" /> <RadioButton style="@style/discount" android:id="@+id/rb_discount9" android:text="@string/label_discount9" /> </RadioGroup>
|
.java文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| for(RadioButton r : rb_firstLine){ r.setOnCheckedChangeListener(this); }
for (RadioButton r : rb_secondLine){ r.setOnCheckedChangeListener(this); } @Override public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) { if (isChecked) { uncheckRadio(buttonView.getId()); } }
private void uncheckRadio(int checkId) { for (RadioButton r : rb_firstLine) { if (r.getId() == checkId) { rg_secondLine.clearCheck(); r.setChecked(true); }else { r.setChecked(false); } } for (RadioButton r : rb_secondLine) { if (r.getId() == checkId) { rg_firstLine.clearCheck(); r.setChecked(true);
}else { r.setChecked(false); } } }
|